O código a seguir pode ser usado para automatizar o Outlook a partir do Excel, Word, Access ou qualquer aplicativo VBA habilitado. Também poderia ser usado em um aplicativo VB6. Ele vai criar uma nova mensagem de e-mail e anexe o arquivo especificado. Em sua forma atual, ele irá exibir essa mensagem para que você verifique antes de clicar em Enviar, no entanto, pode ser facilmente modificado, tal como sugerido no código para enviá-lo imediatamente.
Option ExplicitSub SendMail()Dim olApp As Outlook.ApplicationDim olMail As Outlook.MailItemDim blRunning As Boolean'get applicationblRunning=TrueOn Error Resume NextSet olApp = GetObject(, "Outlook.Application")If olApp Is Nothing ThenSet olApp = New Outlook.ApplicationblRunning=FalseEnd IfOn Error Goto 0Set olMail = olApp.CreateItem(olMailItem)With olMail'Specify the email subject.Subject = "My email with attachment"'Specify who it should be sent to'Repeat this line to add further recipients.Recipients.Add "name@host.com"'specify the file to attach'repeat this line to add further attachments.Attachments.Add "c:\test.txt"'specify the text to appear in the email.Body = "Here is an email"'Choose which of the following 2 lines to have commented out.Display 'This will display the message for you to check and send yourself'.Send ' This will send the message straight awayEnd WithIf Not blRunning Then olApp.QuitSet olApp=NothingSet olMail=NothingEnd Sub
Série de Livros nut Project
Série DONUT PROJECT 2021
Série DONUT PROJECT 2018
Série DONUT PROJECT 2015
Série DONUT PROJECT 2014
Clique aqui e nos contate via What's App para avaliarmos seus projetos
Envie seus comentários e sugestões e compartilhe este artigo!
brazilsalesforceeffectiveness@gmail.com
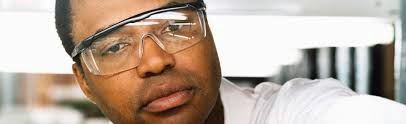
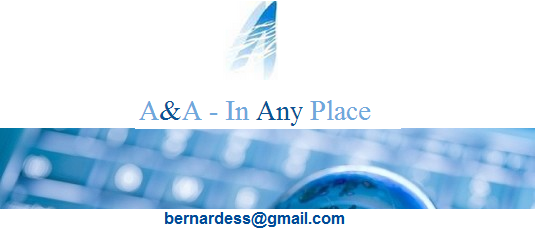